Aug 31, 2006 For information about how to read from a serial port in an asynchronous manner in PowerShell V2, use the DataReceived event on the port object with the Register-ObjectEvent cmdlet. Jeffrey Snover MSFT. Jun 20, 2019 Listens to and read data from a serial port (e.g. DESCRIPTION: The purpose of this script is to keep listening and read data from a serial port. All the data captured will be displayed and log into a file. EXAMPLE./SerialPortReader.ps1 -PortName COM3 -BaudRate 9600. Connecting to read the Serial Port from PowerShell is very easy with few lines of code. However, one problem I have seen is to read a changed value of potentiometer, a new PowerShell session must be started, Not just closing and opening the port. Reading the first setting of the potentiometer: 1. Manage PowerShell from Serial Port Functions to manage PowerShell from Serial Port. It can be used to manage the basic PowerShell commands that are sent to the remote machine via a serial port.
PermalinkJoin GitHub today
GitHub is home to over 40 million developers working together to host and review code, manage projects, and build software together.
Sign upPowershell Serial Port Realtime Dtr Cts
1 contributor
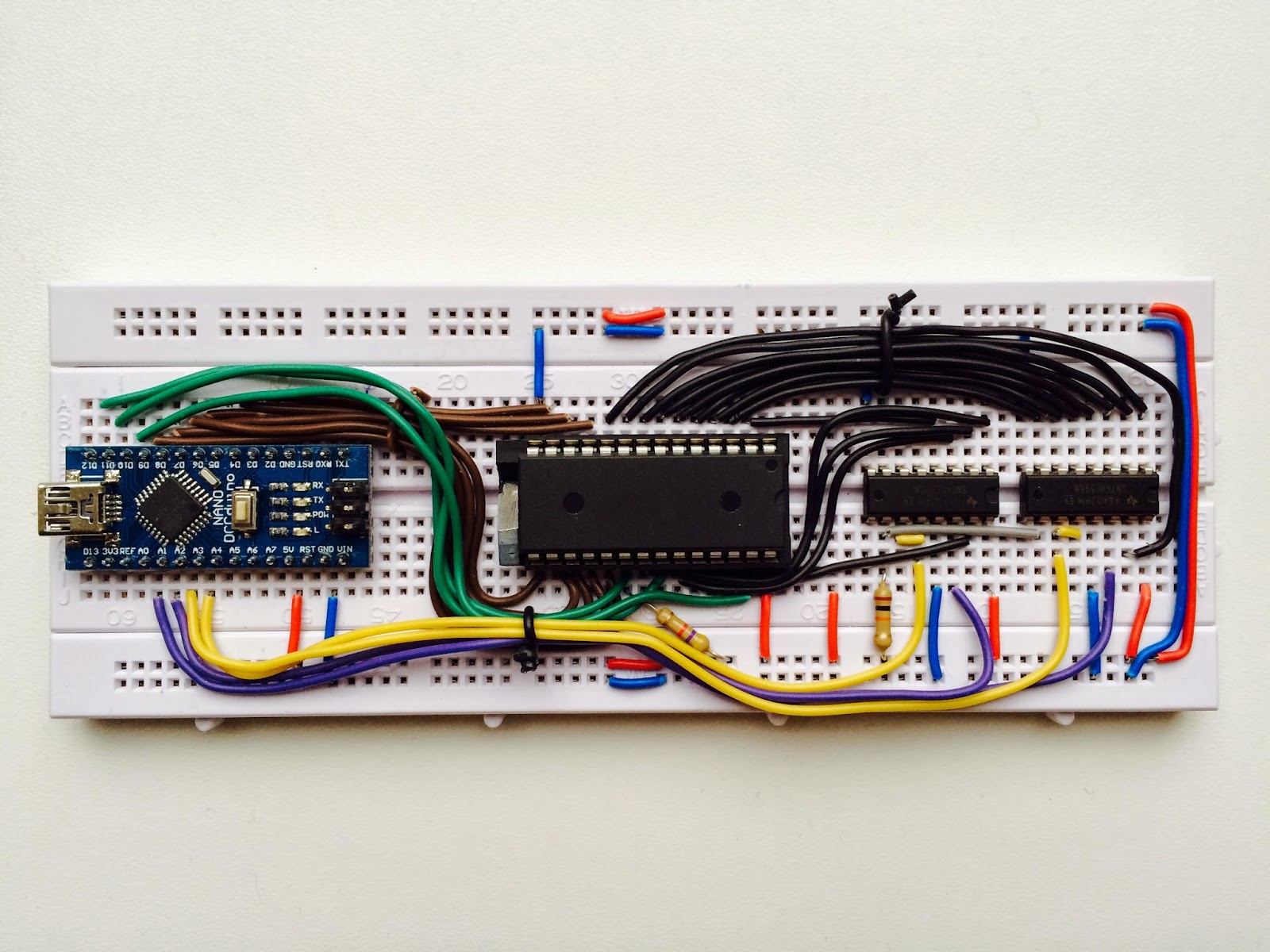
New-Module { |
<# |
.Synopsis |
Create a new serial port to read or write to. |
.DESCRIPTION |
.EXAMPLE |
$myport = New-SerialPort |
Create a new port with a .net event subscribed to the incoming data |
.EXAMPLE |
New-SerialPort -outvariable port -Name COM5 |
Create a new port whislt seing it's configuration. |
BaseStream : |
BaudRate : 9600 |
BreakState : |
BytesToWrite : |
BytesToRead : |
CDHolding : |
CtsHolding : |
DataBits : 8 |
DiscardNull : False |
DsrHolding : |
DtrEnable : False |
Encoding : System.Text.ASCIIEncoding |
Handshake : None |
IsOpen : False |
NewLine : |
Parity : None |
ParityReplace : 63 |
PortName : com5 |
ReadBufferSize : 4096 |
ReadTimeout : 1000 |
ReceivedBytesThreshold : 1 |
RtsEnable : False |
StopBits : One |
WriteBufferSize : 2048 |
WriteTimeout : -1 |
Site : |
Container : |
#> |
functionNew-SerialPort { |
[CmdletBinding()] |
[OutputType([System.IO.Ports.SerialPort])] |
Param |
( |
# Event Identifier |
[Parameter()] |
[ValidatePattern('[a-zA-Z]d')] |
[String] |
$Name='COM3' |
) |
$error.Clear() |
if (Get-Serialport-Name $Name) { |
throw'Name already exists, use Get-SerialPort' |
} |
$port= InstancePort -Name $Name |
if ($port) { |
# Print data only when recieved, No polling the port that's crazy talk. |
Register-ObjectEvent-InputObject $port-EventName 'DataReceived'-SourceIdentifier $Name>$null |
} |
else { |
throw'Port is not defined' |
} |
$port|Write-Output |
} |
functionGet-SerialPort { |
[CmdletBinding()] |
[OutputType([System.IO.Ports.SerialPort])] |
Param |
( |
# Name of port to find, default all |
[Parameter()] |
[string] |
$name='*' |
) |
$real= [System.IO.Ports.SerialPort]::getportnames() | |
ForEach-Object { |
new-object-TypeName PSCustomObject -Property @{ |
'type'='Physical' |
'name'=$psitem.PortName |
'port'=$psitem |
} |
} |
# TODO: implement some kind of virtual port |
# Wrote all of this without testing if you can open a port that doesn't exist in hardware. |
$virtual=Get-EventSubscriber-SourceIdentifier $name-ErrorAction SilentlyContinue | |
Select-Object-ExpandProperty SourceObject | |
ForEach-Object { |
new-object-TypeName PSCustomObject -Property @{ |
'type'='Virtual' |
'name'=$psitem.PortName |
'port'=$psitem |
} |
} |
$real+$virtual|Write-Output |
} |
functionShow-SerialPort { |
[CmdletBinding(DefaultParameterSetName='Name')] |
[OutputType([string])] |
Param |
( |
# Serial Port Object |
[Parameter(ParameterSetName='Object', |
Mandatory=$true, |
Position=0, |
ValueFromPipeline=$true, |
ValueFromPipelineByPropertyName=$true)] |
[System.IO.Ports.SerialPort] |
$Port |
,# Port Name |
[Parameter(ParameterSetName='Name', |
Mandatory=$true, |
Position=0, |
ValueFromPipeline=$true, |
ValueFromPipelineByPropertyName=$true)] |
[string] |
$Name |
) |
if ($Name) { |
$port=Get-SerialPort-Name $Name| |
Where-Object type -eq'Physical'| |
Select-Object-ExpandProperty port |
} |
if (-not$port) { |
throw'No physical port found to open.' |
} |
Write-verbose ('Current port open status: '+$port.isOpen) |
if ( -not$port.isOpen) { $port.open() } |
Write-Verbose'Waiting on data' |
while ($true) { |
Wait-Event-SourceIdentifier $port.portname-Timeout ($port.ReadTimeout/1000) |
} |
# TODO: catch the difference between data or timeout |
# Why does this just loop the same event output |
Write-Output ($port.ReadExisting()) |
} |
functionRemove-SerialPort { |
[CmdletBinding()] |
[OutputType([PSObject])] |
Param |
( |
# Serial port reference |
[Parameter(Position=0, |
Mandatory=$true, |
ValueFromPipeline=$true, |
ValueFromPipelineByPropertyName=$true)] |
[System.IO.Ports.SerialPort[]] |
$port |
,# Remove all events in log |
[switch] |
$clean |
) |
Process { |
Write-Verbose'Stop Listening to data' |
Write-Verbose'$($port.portname) status: $($port.isOpen)' |
$port.Close() |
# Free the resources just to be sure it's safely unbound for sucessive runs of the script |
$port.Dispose() |
# Dispose of the event handler to make sure we're all clean |
Unregister-Event$port.portname-ErrorAction SilentlyContinue |
switch ($clean) { |
$true { |
Remove-Event-SourceIdentifier $port.portName-ErrorAction SilentlyContinue |
} |
Default {} |
} |
Write-Verbose'Event count: $(get-event| measure | select -expandproperty count)' |
} |
} |
functioninstancePort() { |
[CmdletBinding()] |
[OutputType([System.IO.Ports.SerialPort])] |
Param |
( |
# Port Name |
[String] |
$name='COM3' |
,# Baud Rate |
$BaudRate=9600 |
,# Parity Bit |
$ParityBit='None' |
,# Data Bits |
$DataBits=8 |
,# Stop Bit |
$StopBit='one' |
) |
$port=New-Object system.io.ports.serialport $name,$BaudRate,$ParityBit,$DataBits,$StopBit |
$port.ReadTimeout=1000# milliseconds |
Write-Output$port |
} |
Export-ModuleMember'*-*' |
} |
Powershell Serial Connection
Copy lines Copy permalink
Powershell Read Write Serial Port
<# |
.SYNOPSIS |
Listens to and read data from a serial port (e.g. COM port) |
.DESCRIPTION |
The purpose of this script is to keep listening and read data from a serial port. |
All the data captured will be displayed and log into a file. |
.EXAMPLE |
./SerialPortReader.ps1 |
.EXAMPLE |
./SerialPortReader.ps1 -PortName COM3 -BaudRate 9600 |
.EXAMPLE |
./SerialPortReader.ps1 -PortName COM3 -BaudRate 9600 -Parity None -DataBits 8 -StopBits One -Handshake None -OutputFile '.output1.log' -ReadInterval 1000 |
.LINK |
http://heiswayi.github.io |
.AUTHOR |
Heiswayi Nrird, 2016 |
.LICENSE |
MIT |
#> |
[CmdletBinding(SupportsShouldProcess=$true,ConfirmImpact='Medium')] |
[Alias()] |
[OutputType([int])] |
Param |
( |
[Parameter(Mandatory=$true,Position=0)] |
[ValidateNotNullOrEmpty()] |
[string]$PortName='COM1', |
[Parameter(Mandatory=$true,Position=1)] |
[ValidateNotNullOrEmpty()] |
[int]$BaudRate=9600, |
[Parameter(Mandatory=$false,Position=2)] |
[ValidateNotNullOrEmpty()] |
[System.IO.Ports.Parity]$Parity= [System.IO.Ports.Parity]'None', |
[Parameter(Mandatory=$false,Position=3)] |
[ValidateNotNullOrEmpty()] |
[int]$DataBits=8, |
[Parameter(Mandatory=$false,Position=4)] |
[ValidateNotNullOrEmpty()] |
[System.IO.Ports.StopBits]$StopBits= [System.IO.Ports.StopBits]'One', |
[Parameter(Mandatory=$false,Position=5)] |
[ValidateNotNullOrEmpty()] |
[System.IO.Ports.Handshake]$Handshake= [System.IO.Ports.Handshake]'None', |
[Parameter(Mandatory=$false,Position=6)] |
[ValidateNotNullOrEmpty()] |
[string]$OutputFile='notempty', |
[Parameter(Mandatory=$false,Position=7)] |
[ValidateNotNullOrEmpty()] |
[int]$ReadInterval=1000 |
) |
<# |
.SYNOPSIS |
Listens to and read data from a serial port (e.g. COM port) |
.DESCRIPTION |
The purpose of this script is to keep listening and read data from a serial port. |
All the data captured will be displayed and log into a file. |
.EXAMPLE |
SerialPortReader |
.EXAMPLE |
SerialPortReader -PortName COM3 -BaudRate 9600 |
.EXAMPLE |
SerialPortReader -PortName COM3 -BaudRate 9600 -Parity None -DataBits 8 -StopBits One -Handshake None -OutputFile '.output1.log' -ReadInterval 1000 |
.LINK |
http://heiswayi.github.io |
.AUTHOR |
Heiswayi Nrird, 2016 |
.LICENSE |
MIT |
#> |
functionSerialPortReader |
{ |
[CmdletBinding(SupportsShouldProcess=$true,ConfirmImpact='Medium')] |
[Alias()] |
[OutputType([int])] |
Param |
( |
[Parameter(Mandatory=$true,Position=0)] |
[ValidateNotNullOrEmpty()] |
[string]$PortName='COM1', |
[Parameter(Mandatory=$true,Position=1)] |
[ValidateNotNullOrEmpty()] |
[int]$BaudRate=9600, |
[Parameter(Mandatory=$false,Position=2)] |
[ValidateNotNullOrEmpty()] |
[System.IO.Ports.Parity]$Parity= [System.IO.Ports.Parity]'None', |
[Parameter(Mandatory=$false,Position=3)] |
[ValidateNotNullOrEmpty()] |
[int]$DataBits=8, |
[Parameter(Mandatory=$false,Position=4)] |
[ValidateNotNullOrEmpty()] |
[System.IO.Ports.StopBits]$StopBits= [System.IO.Ports.StopBits]'One', |
[Parameter(Mandatory=$false,Position=5)] |
[ValidateNotNullOrEmpty()] |
[System.IO.Ports.Handshake]$Handshake= [System.IO.Ports.Handshake]'None', |
[Parameter(Mandatory=$false,Position=6)] |
[ValidateNotNullOrEmpty()] |
[string]$OutputFile='notempty', |
[Parameter(Mandatory=$false,Position=7)] |
[ValidateNotNullOrEmpty()] |
[int]$ReadInterval=1000 |
) |
$proceed=$false |
Write-Output ('Checking PortName...') |
foreach ($itemin [System.IO.Ports.SerialPort]::GetPortNames()) |
{ |
if ($item-eq$PortName) |
{ |
$proceed=$true |
Write-Output ('--> PortName '+$PortName+' is available') |
break |
} |
} |
if ($proceed-eq$false) |
{ |
Write-Warning ('--> PortName '+$PortName+' not found') |
return |
} |
$filename='' |
try |
{ |
$port=New-Object System.IO.Ports.SerialPort |
$port.PortName=$PortName |
$port.BaudRate=$BaudRate |
$port.Parity=$Parity |
$port.DataBits=$DataBits |
$port.StopBits=$StopBits |
$port.Handshake=$Handshake |
$currentDate=Get-Date-Format yyyyMMdd |
$fileExt='.log' |
if ($OutputFile-eq'notempty') |
{ |
$filename= (Get-Item-Path '.'-Verbose).FullName +'SerialPortReader_'+$currentDate+$fileExt |
} |
else |
{ |
$filename=$OutputFile |
} |
Write-Output ('Establishing connection to the port...') |
Start-Sleep-Milliseconds 1000 |
$port.Open() |
Write-Output$port |
Write-Output ('--> Connection established.') |
Write-Output ('') |
} |
catch [System.Exception] |
{ |
Write-Error ('Failed to connect : '+$_) |
$error[0] |Format-List-Force |
if ($port-ne$null) { $port.Close() } |
exit1 |
} |
Start-Sleep-Milliseconds 1000 |
do |
{ |
$key=if ($host.UI.RawUI.KeyAvailable) { $host.UI.RawUI.ReadKey('NoEcho, IncludeKeyDown') } |
if ($port.IsOpen) |
{ |
Start-Sleep-Milliseconds $ReadInterval |
#$data = $port.ReadLine() |
$data=$port.ReadExisting() |
<# |
[byte[]]$readBuffer = New-Object byte[] ($port.ReadBufferSize + 1) |
try |
{ |
[int]$count = $port.Read($readBuffer, 0, $port.ReadBufferSize) |
[string]$data = [System.Text.Encoding]::ASCII.GetString($readBuffer, 0, $count) |
} |
catch { } |
#> |
$length=$data.Length |
# remove newline chars |
$data=$data-replace [System.Environment]::NewLine,'' |
$getTimestamp=Get-Date-Format yyyy-MM-dd HH:mm:ss.fff |
Write-Output ('['+$getTimestamp+'] '+$data) |
if ($length-gt0) { ExportToFile -Filename $filename-Timestamp $getTimestamp-Data $data } |
} |
} until ($key.VirtualKeyCode-eq81) # until 'q' is pressed |
if ($port-ne$null) { $port.Close() } |
} |
<# |
.SYNOPSIS |
Listens to and read data from a serial port (e.g. COM port) |
.DESCRIPTION |
The purpose of this script is to keep listening and read data from a serial port. |
All the data captured will be displayed and log into a file. |
.EXAMPLE |
ExportToFile -Filename <fullpath> -Timestamp <time string> -Data <data string> |
.AUTHOR |
Heiswayi Nrird, 2016 |
.LICENSE |
MIT |
#> |
functionExportToFile |
{ |
[CmdletBinding(SupportsShouldProcess=$true,ConfirmImpact='Medium')] |
[OutputType([string])] |
Param |
( |
[Parameter(Mandatory=$true,Position=0)] |
[string]$Filename, |
[Parameter(Mandatory=$true,Position=1)] |
[string]$Timestamp, |
[Parameter(Mandatory=$true,Position=2)] |
[string]$Data |
) |
try |
{ |
'['+$Timestamp+'] '+$Data|Out-File-FilePath $Filename-Encoding ascii -Append |
} |
catch [System.Exception] |
{ |
Write-Warning ('Failed to export captured data : '+$_) |
return |
} |
} |
if (-not ($myinvocation.line.Contains('`$here`$sut'))) { |
SerialPortReader -PortName $PortName-BaudRate $BaudRate-Parity $Parity-DataBits $DataBits-StopBits $StopBits-Handshake $Handshake-OutputFile $OutputFile-ReadInterval $ReadInterval |
} |
commented Nov 1, 2018
I have an Error in Line 201 Results in PS: Fix (use ' in Format-String): |